Windows Azure storage gives you several persistent and durables storage options. In this blog post I want to look at Table storage (which I prefer to call Entity storage because you can store any mix of entities in these tables; so you can store products AND customers in the same table). For this walkthrough you’ll need the AZURE SDK and setup for development…
1. Getting ready
Start Visual Studio 2010 and create a new Azure project called MessageServiceWithTables:
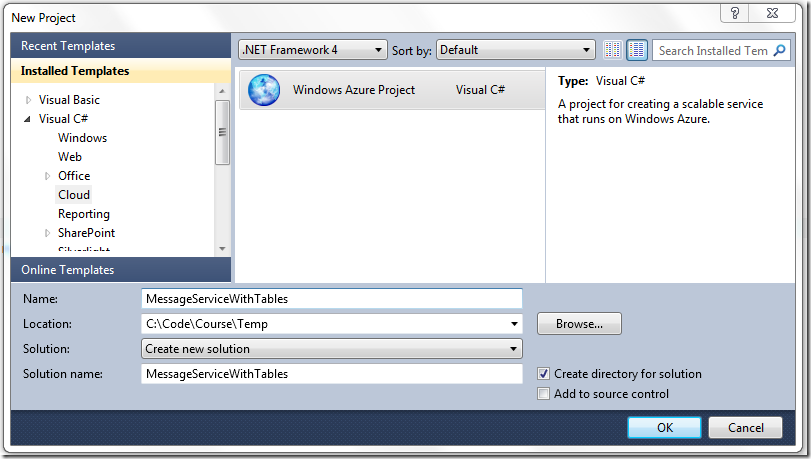
In the New Windows Azure Project dialog select the ASP.NET Web Role and press the > button, then rename the project to CloudMessages:
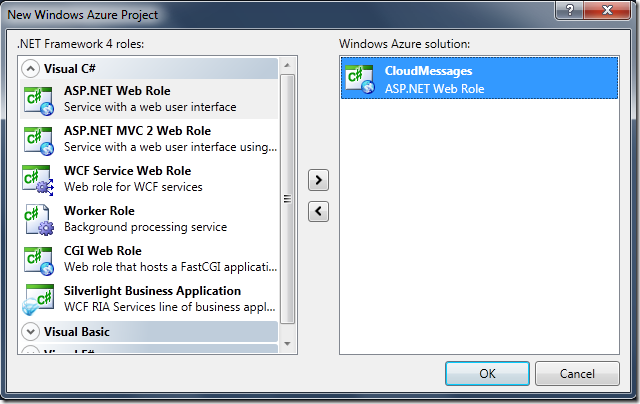
Replace the content of default.aspx with the following:
<%@ Page Title="Home Page" Language="C#" MasterPageFile="~/Site.master" AutoEventWireup="true"
CodeBehind="Default.aspx.cs" Inherits="CloudMessages._Default" %>
<asp:Content ID="HeaderContent" runat="server" ContentPlaceHolderID="HeadContent">
</asp:Content>
<asp:Content ID="BodyContent" runat="server" ContentPlaceHolderID="MainContent">
<p>
<asp:TextBox ID="messageText" runat="server" Width="396px"></asp:TextBox>
<asp:Button ID="postButton" runat="server" OnClick="postButton_Click" Text="Post message" />
</p>
</asp:Content>
2. Creating the table store entity classes
Add a new Class Library project to your solution, and call it MessagesLib. Delete class1.cs. Add a new class called MessageEntity.
2.1 Creating the entity class
We want to derive this class from TableServiceEntity, but first we need to add a couple of references. So select Add Reference… on the library project.
Browse to Program Files\Windows Azure SDK\v1.4\ref and select following libraries (or simply select them all):
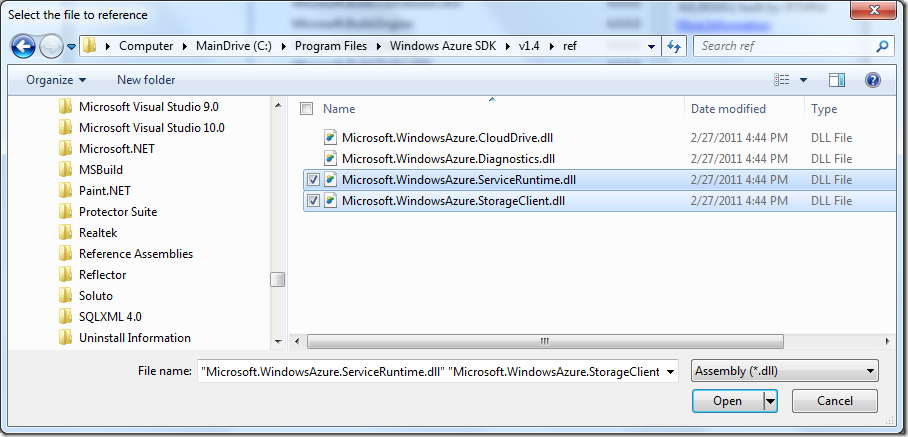
You also need to add a reference to System.Data.Services.Client (I’m using Power Tools, so the Add Reference looks different (excuse me, better!):
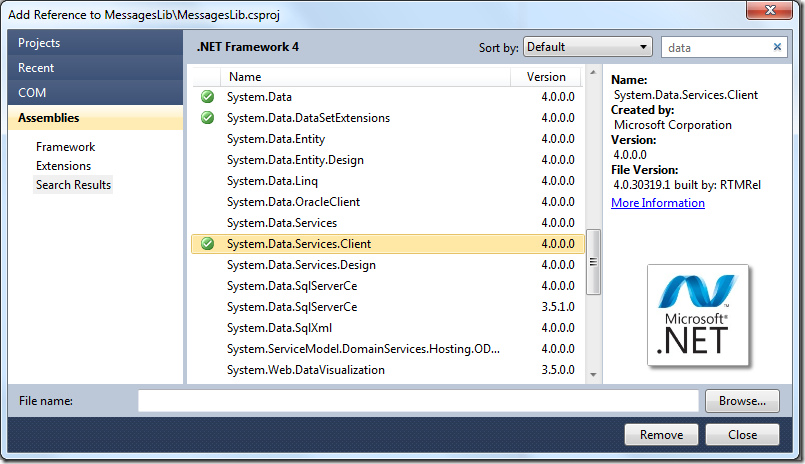
Now you’re ready to add the MessageEntity class deriving from the TableServiceEntity base class.
public class MessageEntity : TableServiceEntity
{
public MessageEntity() { }
public MessageEntity(string partitionKey, string rowKey, string message)
: base( partitionKey, rowKey )
{
Message = message;
}
public string Message { get; set; }
}
This base class has three properties used by table storage: the partition key, the row key and the timestamp:
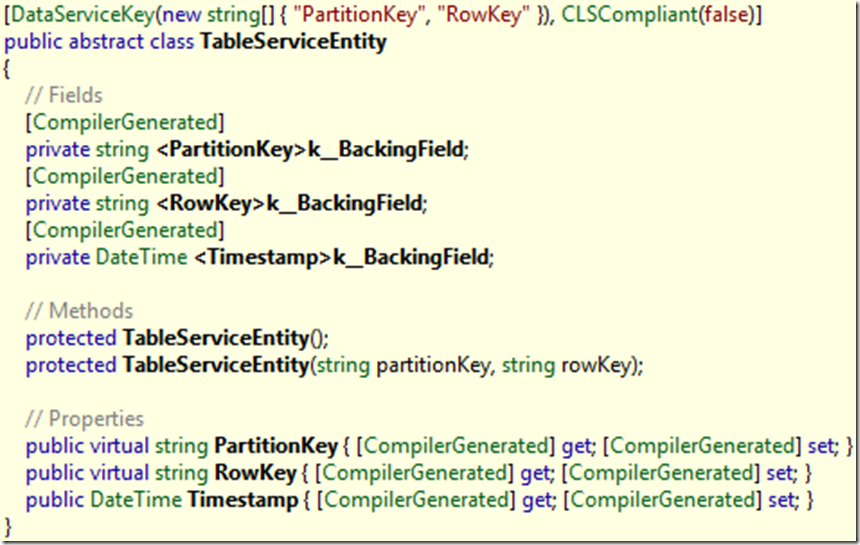
The partition key is used as follows: all entities sharing the same partition key share the same storage device, they are kept together. This make querying these objects faster. But on the other hand, entities with different partition keys can be stored on different machines, allowing queries to be distributed over these machines when there are many instances. So choosing the partition key is a tricky thing, and there are no automated tools to help you here. Some people will use buckets (like from 0 to 9) and evenly distribute their instances over all buckets.
The row key makes the entity unique and the timestamp is used for concurrency checking (optimistic concurrency).
So, what do we need to store? Since we just want to store messages we add a single Message property and constructors for easy instantiation.
In the next blog post we’ll be looking at creating the table in table storage and inserting new data…