I’m currently playing with Azure and the Azure training kit, and I learned something cool today. When you work with Azure you can setup multiple worker roles for your Azure application. If you want to make these roles talk to one another you can use the queuing mechanism which is part of Azure. But you can also use WCF dual interface mechanism. Imagine you want to build a chat application using Azure and WCF. In this case you define a worker role that exposes a dual interface like this:
Code Snippet
- [ServiceContract(
- Namespace = "urn:WindowsAzurePlatformKit:Labs:AzureTalk:2009:10",
- CallbackContract = typeof(IClientNotification),
- SessionMode = SessionMode.Required)]
- public interface IChatService
- {
- /// <summary>
- /// Called by client to announce they are connected at this chat endpoint.
- /// </summary>
- /// <param name="userName">The user name of the client.</param>
- /// <returns>The ClientInformation object for the new session.</returns>
- [OperationContract(IsInitiating = true)]
- ClientInformation Register(string userName);
-
- /// <summary>
- /// Sends a message to a user.
- /// </summary>
- /// <param name="message">The message to send.</param>
- /// <param name="sessionId">The recipient's session ID.</param>
- [OperationContract(IsInitiating = false)]
- void SendMessage(string message, string sessionId);
-
- /// <summary>
- /// Returns a list of connected clients.
- /// </summary>
- /// <returns>The list of active sessions.</returns>
- [OperationContract(IsInitiating = false)]
- IEnumerable<ClientInformation> GetConnectedClients();
- }
The IClientNotification interface is the call-back interface which is implemented by the client of the service. The client calls the Register method on the server, which then can keep track of the client by using the callback interface:
Code Snippet
- IClientNotification callback =
- OperationContext.Current.GetCallbackChannel<IClientNotification>();
If you host the service as a single instance, all clients will register to the same service, so this service can easily track each client. But if you grow and start using multiple instances of your service in Azure, each client will register to a single instance, so each instance will know only about its own clients. If two clients, registered to different instances, want to communicate, the services will have to handle the communication for this. The solution is easy, make them also expose the IClientNotification callback service interface using internal endpoints, so they can communicate to each other:
Code Snippet
- public class ChatService : IChatService, IClientNotification
Of course each service will have to be able to find the other service instances. This you can do with the RoleEnvironment class, which you can use in your worker role class:
Code Snippet
- var current = RoleEnvironment.CurrentRoleInstance;
- var endPoints = current.Role.Instances
- .Where(instance => instance != current)
- .Select(instance => instance.InstanceEndpoints["NotificationService"]);
This does require the worker role to define an internal endpoint. You can do this in Visual Studio 2010. Select the worker role’s properties, go to the endpoint tab and enter an internal endpoint:
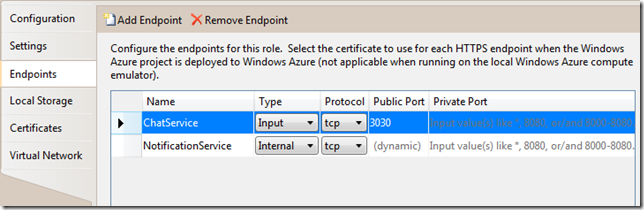
The rest of the code is straightforward (of your comfortable with WCF that is) and can be found in the Azure Training Kit (look for the Worker Role Communication lab).