Setting up a Static Web App
A nice addition to Azure is static web apps. The idea is not really new, but the implementation is new, and nice. (Beware: still in preview) What is the idea ? Modern webapplications focus heavily on doing things in the client, using javascript. In the past we used server-side code fpr generating and controlling the UI, but, luckily, those day lay behind us. In some cases we still create the initial UI server-side, after which javascript will do the UI updates. In some cases the entire UI will be created using javascript frameworks like react, vue, blazor, etc... The only reason why we still use server-side code is for writing REST services, which can be called from the client-side.
The word "static" here refers to all static files that we need, like javascript, css, html, etc... We typically host these on a web server. But using Azure, we could also put them in public blob storage and et them from there. We could put Azure CDN in front of the blob storage, so you could get those files from the nearest geographical location. And for the REST services we could go completely serverless by using Azure Functions. Brilliant !
And that is exactly what Static Web Apps are doing, packaged into one Azure Resource.
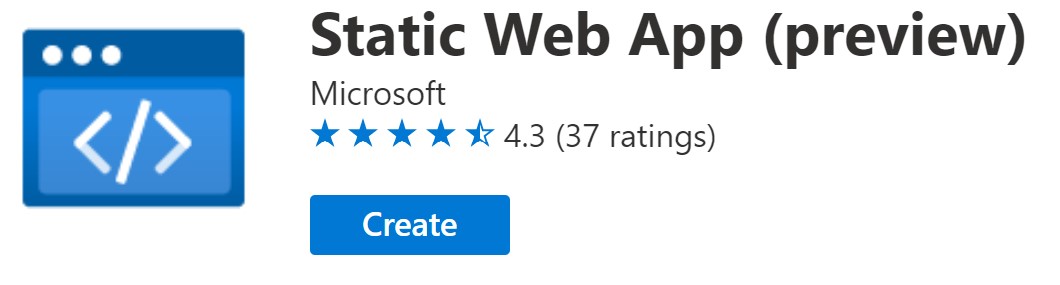
Beware: the product is still in preview, but I already love it. I did a few test with simple html-javascript apps. Time for doing some things with React.
The idea is that whenever you do a push in GitHub or Azure DevOps, your application starts building, and will be deployed to azure.
So I created this basic "Hello World" React application. I build the output using npx, which creates a build folder with the necessary files. That is what needs to be deployed in azure.
You will need to install the Azure Static Web Apps extension to Visual Studio Code. This allows you to set up a static web app. It can also be done from within the portal, but I prefer this way. When creating a new Static Web App from within VS Code, you'll have to set up a few defaults, like the javascript framework you'll use, etc... If the framework you use is not listed, you can still configure it manually. What do you need to configure:
- What GitHub repository will contain your project
- Name of the static web app
- Default framework
- location of your application code
- location where you will put your azure functions.
- And the location of your build output
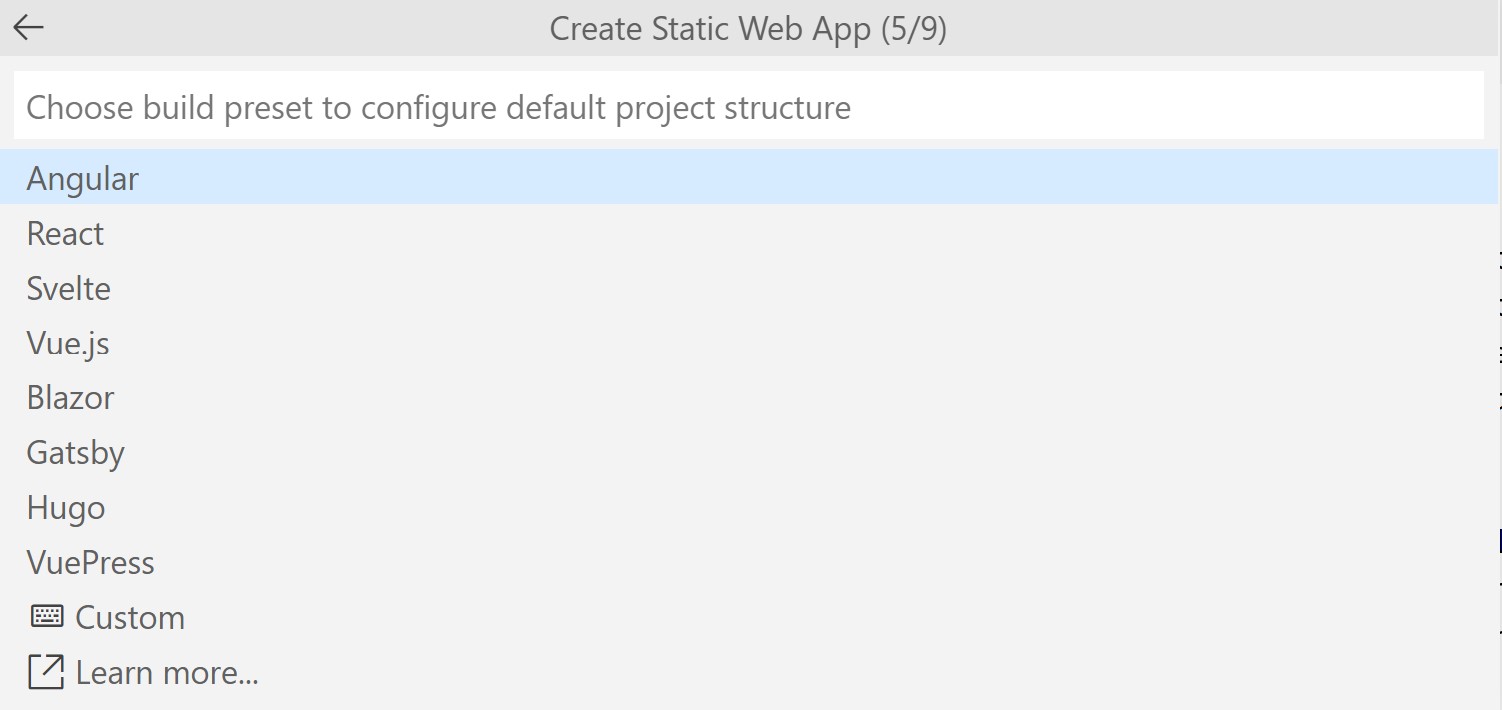
This will create a static web app in azure, and create a workflow in your github repository for building and deploying to azure, eveytime when you push your changes.
Adding Services
Adding Services can be done using Azure Functions. Next to the + Button for creating a new Static Web App, we have a button for creating a new function.

This allows you to create a new Http-triggered Function in Javascript, Typescript, Python or C#. Let's create something super simple in the api folder:
[FunctionName("SimpleFunction")]
public static async Task<IActionResult> Run(
[HttpTrigger(AuthorizationLevel.Anonymous, "get",Route = null)] HttpRequest req,
ILogger log)
{
return new OkObjectResult("well done, my young apprentice");
}
Calling it from within your client-side code is as usual:
async function getMessage() {
let response = await fetch('/api/simplefunction');
let txt = await response.text();
return txt;
}
Remark that, after deployment, you do not get a separate Function App. The Function appears as part of your static web app.
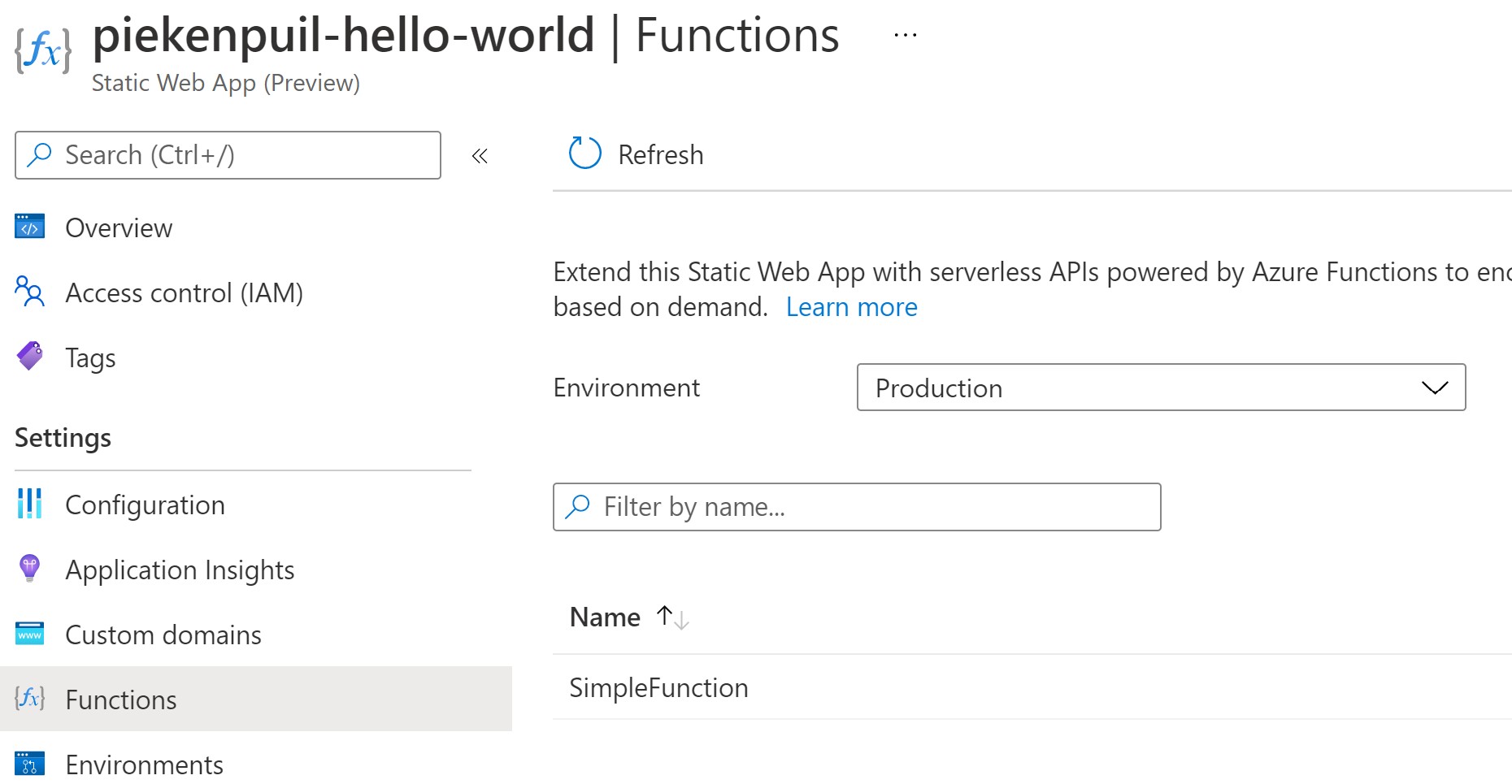
Test Versions
Also a nice feature is that after creating a pull request, you get an additional URL for the branch on which you created the pull request. Which, of course, allows you to test it before you merge the branch.
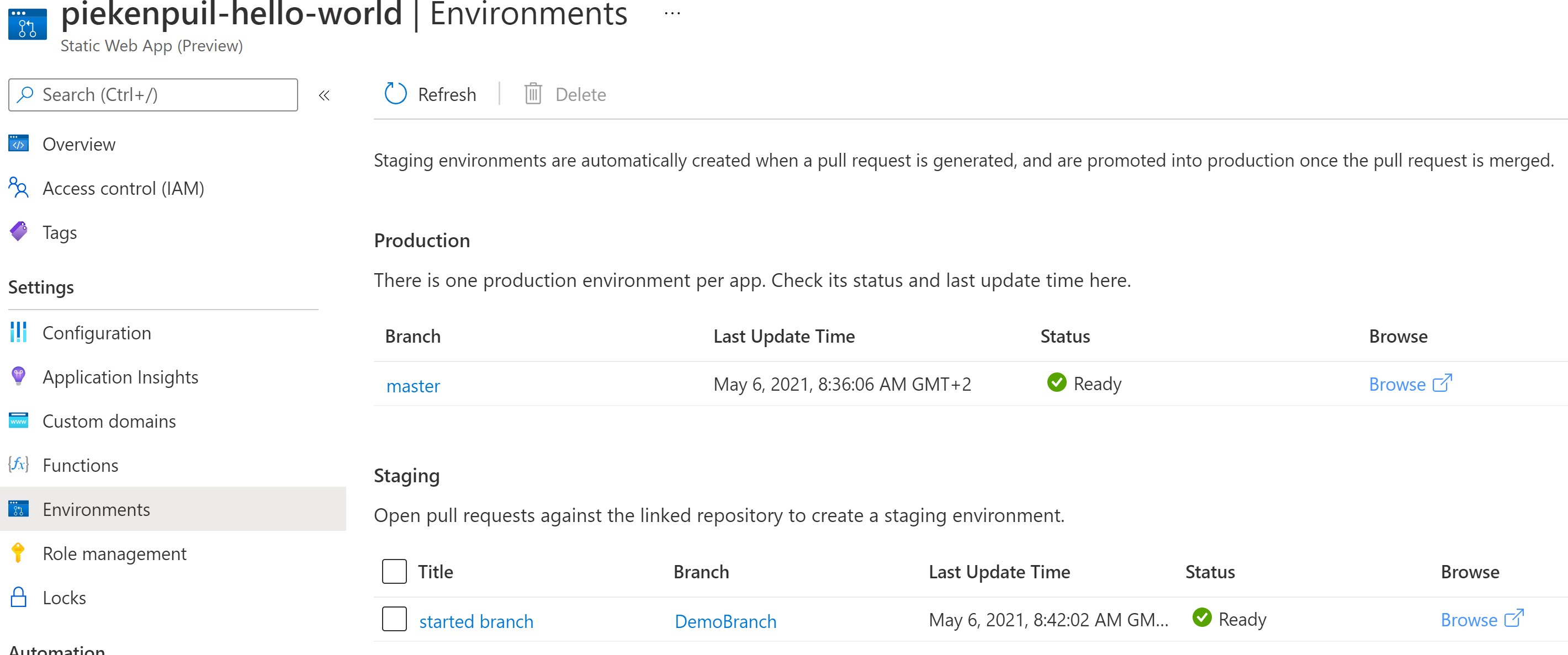