At last, part 2 of this blogpost. In part 1, we created an updatable Live Tile. We still need to update it with a Background task. We start by adding a Windows Phone Scheduled Task Agent, which is a projecttype added with the devtools for the Mango-version. This type allows you to ask tasks to be performed in the background. By default the task will run every 30 minutes (It can take longer depending on the memorypressure of the device). In here you can have an override of OnInvoke, that should perform the actual backgroundtask of your app.
The control (Indicator) whom I am using for creating a WriteableBitmap with, needs to be accessible from within the app ánd from within the backgroundtask. Since the app already needs a reference to the BackgroundTask, we cannot create a reference in the opposite direction. So we need to move the Indicator into a separate class-library.
You’ll probably see that this works quiet nicely, until all of a sudden your solution no longer builds. In your code all of the Indicator-properties seems to be absent. I presume it’s a bug in Visual Studio, but from time to time the BuildAction-property for Indicator.xaml changes, which prevents your solution from building. If it happens to you: change it back to “Page” :
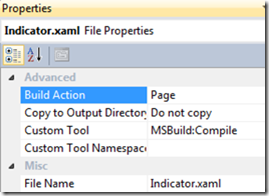
Let’s do a little update of the tile we created :
1: Deployment.Current.Dispatcher.BeginInvoke(() =>
2: {
3: Indicator indicator = new Indicator();
4:
5: indicator.KPI1Brush = new SolidColorBrush(Colors.Magenta);
6: indicator.KPI2Brush = new SolidColorBrush(Colors.Orange);
7: indicator.KPI3Brush = new SolidColorBrush(Colors.Red);
8: indicator.KPI4Brush = new SolidColorBrush(Colors.Yellow);
9:
10: indicator.Measure(new Size(173, 173));
11: indicator.Arrange(new Rect(0, 0, 173, 173));
12:
13:
14: string indic = "/Shared/ShellContent/indicator.jpg";
15: WriteableBitmap bm = new WriteableBitmap(indicator, null);
16: IsolatedStorageFile isolated = IsolatedStorageFile.GetUserStoreForApplication();
17: if (isolated.FileExists(indic))
18: {
19: isolated.DeleteFile(indic);
20: }
21: IsolatedStorageFileStream stream = isolated.CreateFile(indic);
22: bm.SaveJpeg(stream, 50, 50, 0, 100);
23: stream.Flush();
24: stream.Close();
25:
26: ShellTile tile = ShellTile.ActiveTiles.FirstOrDefault();
27: ShellTileData data = new StandardTileData()
28: {
29: BackContent = "Sober at 18:23",
30: BackBackgroundImage = new Uri("isostore:/Shared/ShellContent/indicator.jpg", UriKind.Absolute),
31: BackTitle = "0.123"
32: };
33:
34: tile.Update(data);
35: });
This is of course very similar to the code in the first part of this blogpost. You can see that in here we also store the bitmap in isolated storage. The nice thing here is that the isolated storage is being shared between the backgroundtask, and the application for which you create the task. So it could also be used for transferring data from the app to the task.
Actually we’re changing stuff on the GUI Thread from a background thread, that’s why we need the dispatcher-object here.
One little problem remains : I would like to set one of the KPI-colors of my indicator to the PhoneForeGroundColor. In the app I do as such :
new SolidColorBrush((Color)App.Current.Resources["PhoneForegroundColor"]);
(Or use “PhoneForegroundBrush” for getting a Brush-object) But what to do in the BackgroundTask, since I do not have access to the App-class in there ? No problem: you can also access the system-resources through objects inheriting from FrameworkElement :
new SolidColorBrush((Color) indicator.Resources["PhoneForegroundColor"]);
Cool: ready to deploy !