This article shows step by step how to connect a side-loaded modern app to a legacy component via the “Brokered Windows Runtime Components for Side-Loaded applications” feature. This feature comes with Windows 8.1 Update and allows Modern apps to break outside the AppContainer and connect to desktop components such as legacy .NET class libraries. This gives the side-loaded app direct access to native platform capabilities, including COM Interop and P/Invoke. For a detailed introduction I would encourage you to take a look at this Build 2014 session by @devhawk and read this MSDN white paper. A Brokered Windows Runtime Component is a new type of component that bridges the technology gap between the Windows Runtime and the .NET world. It allows you to write a touch optimized modern Store app against legacy components that sit on the same desktop. As an example I created a legacy application with a data access layer DLL that uses SQL Server Express and Linq-to-SQL, and a Windows forms executable:
Here’s the code from the data access layer that fetches the employees from the Northwind database (for the fans: the SQL script to create that database is in the source code of the attached project):
public static Dto.Employee[] GetAllEmployees()
{
using (NorthwindDataContext northWind = new NorthwindDataContext())
{
var query = from e in northWind.Employees
select new Dto.Employee()
{
Name = e.FirstName + " " + e.LastName,
Title = e.Title,
BirthDate = e.BirthDate,
Picture = e.Photo.ToArray()
};
return query.ToArray();
}
}
A combination of a Brokered Windows Runtime Component and a Brokered Windows ProxyStub allowed me to reuse the data access layer of that legacy application in a modern app:
I tried for a couple of hours to build and link the necessary components the manual way, as explained in the White Paper. But I’m not really skilled in building Runtime Components, and the last time I saw the internals of a C++ project was a very long time ago in a galaxy far away. When I was about to give up, the Brokered WinRT Component Project Templates for Visual Studio were released. And this is what happened then:
I installed the templates:
Then I added a brokered component to the solution:
The Brokered Component is the component to which the side-loaded app talks. It’s a hybrid component that can get a reference to the legacy data access layer, but all its public members should be Windows Runtime compatible. This overview of Windows Runtime base data types came in handy. It’s probably obsolete in this particular sample app, but I did a bit of massaging the data. In a production application this would be the main responsibility of the Brokered Component:
public Employee[] GetAllEmployees()
{
var dbe = Dal.GetAllEmployees();
var result = new List<Employee>();
foreach (var item in dbe)
{
result.Add(new Employee() { Name = item.Name, Title = item.Title, Age = Age(item.BirthDate) });
}
return result.ToArray();
}
The side-loaded app can not directly talk to the Brokered Component since it’s targeting a different framework family. The following happens when you try this out:
There needs to be a so called Brokered ProxySub in between the Modern app and the Brokered Windows Runtime Component. Fortunately there is a template for this:
The ProxyStub project is preconfigured for its task and is populated by making a reference to the Brokered Runtime Component:
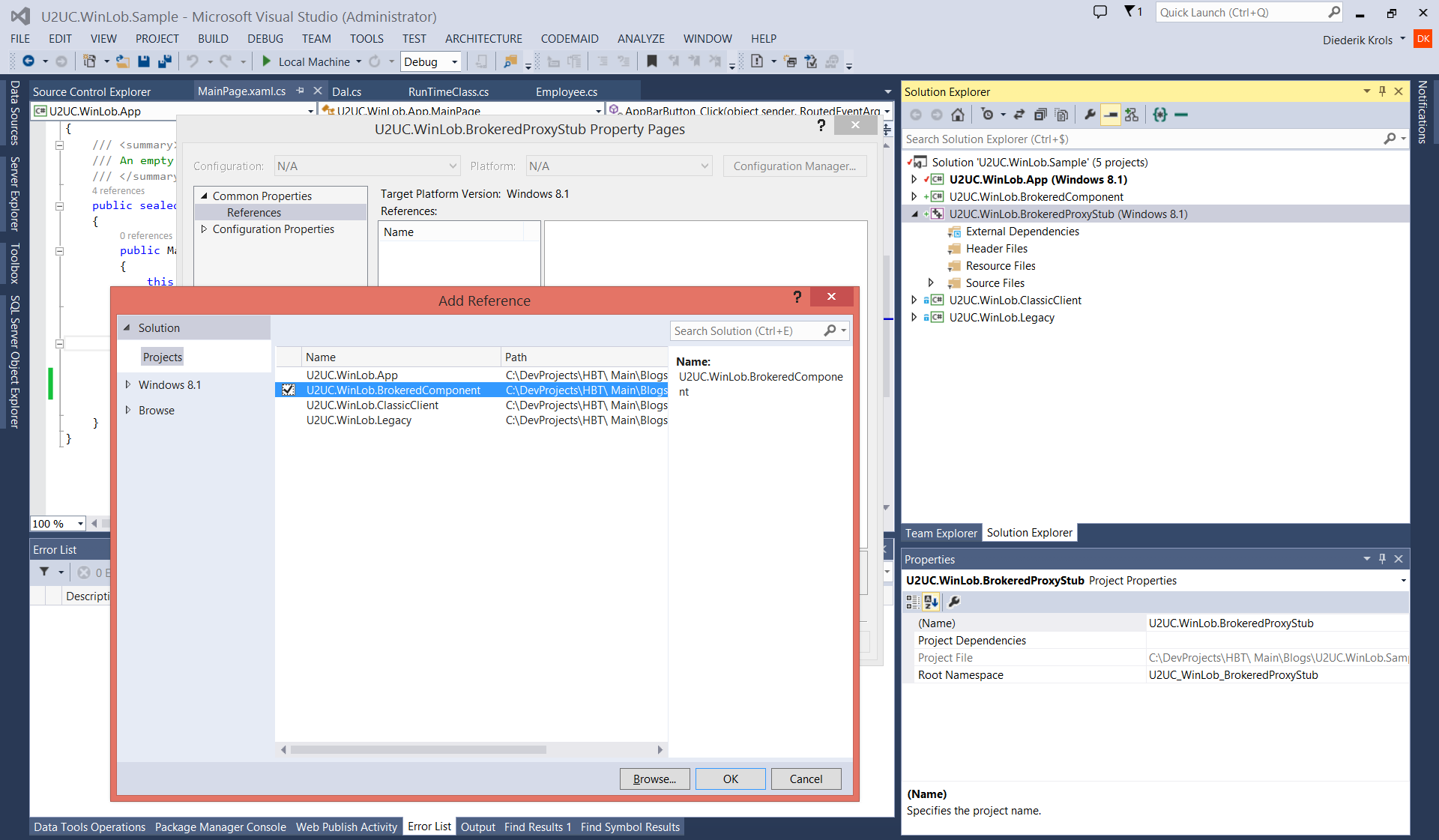
Before the side-loaded app can use it, the ProxyStub needs to be registered as a COM-component. This task gets automated if you run Visual Studio as an Administrator, and configure the Linker:
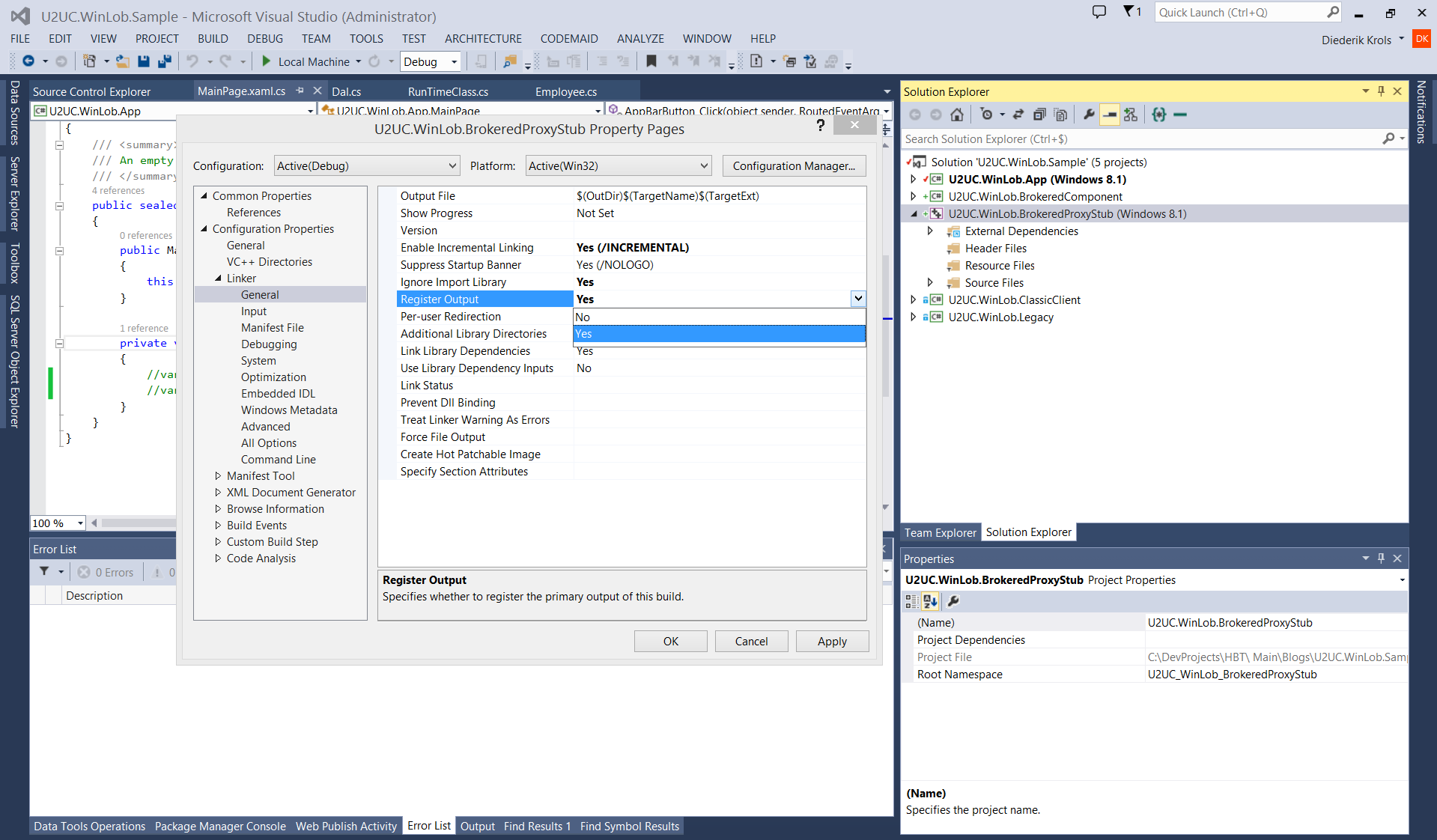
The Modern app needs to make a reference to the ProxyStub:
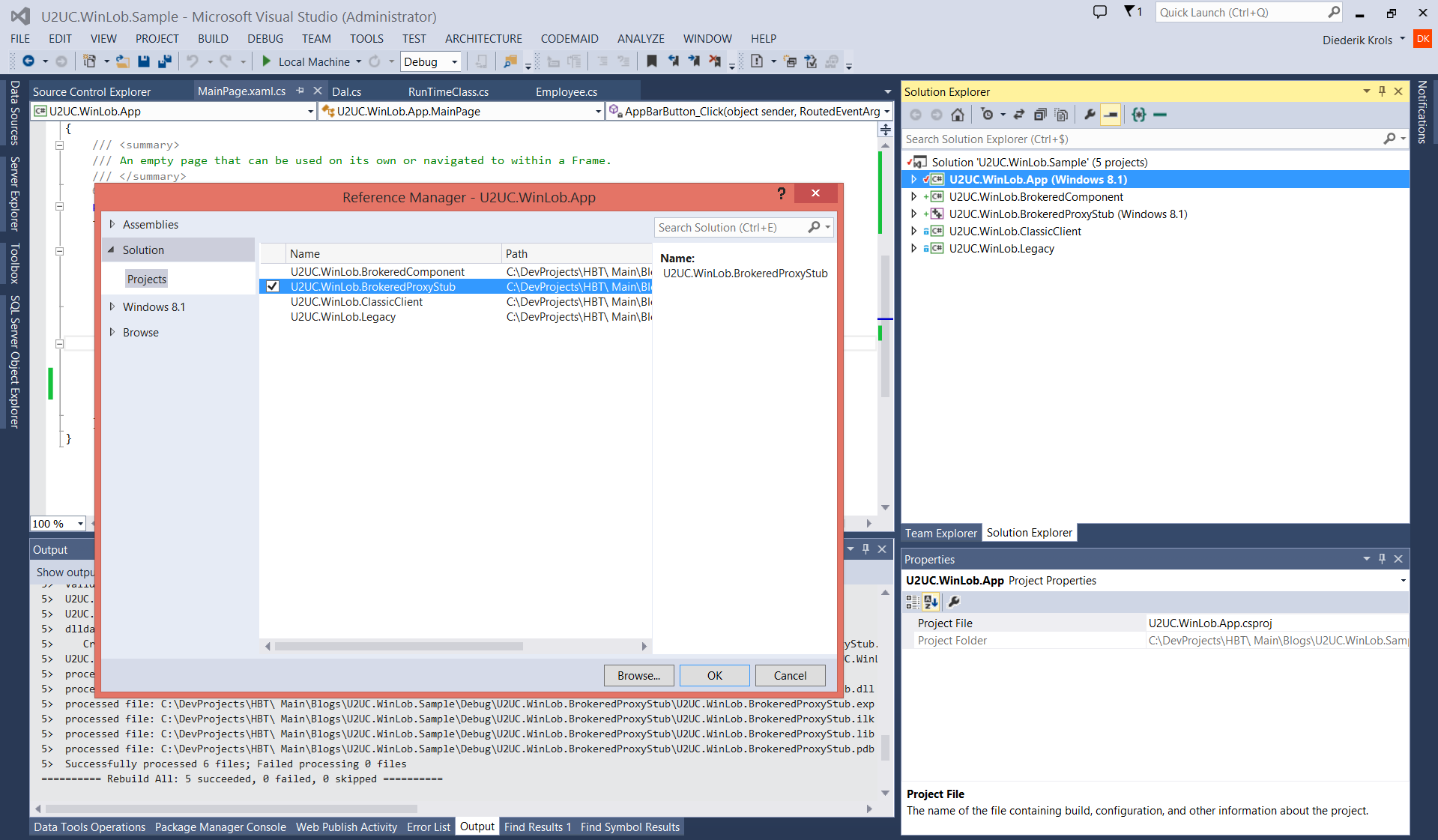
The side-loaded app now has access to the Brokered Component (no MVVM here since I wanted to keep this sample as straightforward as possible):
private void AppBarButton_Click(object sender, RoutedEventArgs e)
{
var broker = new U2UC.WinLob.BrokeredComponent.RunTimeClass();
var data = broker.GetAllEmployees();
this.Employees.ItemsSource = data;
}
If you run the app now, it still goes wrong:
You have to make sure that the fully qualified name of the Brokered Component together with its path are defined in the app’s manifest:
Brokered Windows Runtime Components will enable the development of a new breed of corporate line-of-business apps: touch-first modern apps that are connected to existing enterprise data and processes.
Here’s the sample app, it was written with Visual Studio 2013 Update 2 (RC) for Windows 8.1 Update. If you want to play with it, don’t forget to run as Adminstrator and change the path in the app’s manifest: U2UC.WinLob.Sample.zip (1.2MB)
Enjoy!
XAML Brewer