This article demonstrates how to databind a radiobutton to an enumeration element, in a WPF application. The MVVM viewmodel has a property of an enumeration type, which is bound to the IsChecked property of the radiobutton. A value converter compares the value from the viewmodel with an enumeration element that is provided as parameter to the converter. The Convert method returns true if both match, the ConvertBack method updates the bound value if there's no match.
Here's a screenshot of the attached sample application. The radiobuttons are bound to the Reindeer property -an enumeration- of the viewmodel. The Vote-button updates that same property through a command:
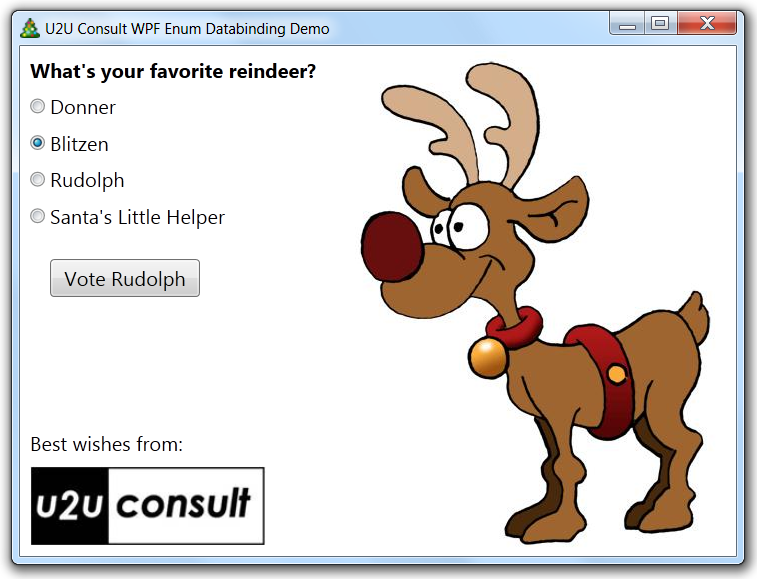
Here's the converter:
namespace U2UConsult.WPF.Converters
{
using System;
using System.Globalization;
using System.Windows.Data;
/// <summary>
/// Converts an enum to a boolean.
/// </summary>
public class EnumToBooleanConverter : IValueConverter
{
/// <summary>
/// Compares the bound value with an enum param. Returns true when they match.
/// </summary>
public object Convert(object value, Type targetType, object param, CultureInfo culture)
{
return value.Equals(param);
}
/// <summary>
/// Updates the bound value if it's different from the parameter.
/// </summary>
public object ConvertBack(object value, Type targetType, object param, CultureInfo culture)
{
return (bool)value ? param : Binding.DoNothing;
}
}
}
In your application, all you need to do is create an enumeration:
public enum Reindeer
{
Donner,
Blitzen,
Rudolph,
SantasLittleHelper
}
In the viewmodel, create a property of the enumeration type:
private Reindeer reindeer;
public event PropertyChangedEventHandler PropertyChanged;
public Reindeer Reindeer
{
get { return reindeer; }
set {
reindeer = value;
this.PropertyChanged.Raise(this, o => o.Reindeer);
}
}
The propertychanged notification makes sure that the radiobutton follows all modifications of the property.
In the view, define the converter instance as a resource in XAML:
<Window.Resources>
<cv:EnumToBooleanConverter x:Key="EnumToBooleanConverter" />
</Window.Resources>
Bind the viewmodel's property to the Ischecked property of each radioButton. The enumeration value is provided as parameter to the converter. Don't use the string representation of the element's value, but use the x:Static markup extension:
<RadioButton Content="Donner"
IsChecked="{Binding Reindeer,
Converter={StaticResource EnumToBooleanConverter},
ConverterParameter={x:Static vm:Reindeer.Donner}}" />
<RadioButton Content="Blitzen"
IsChecked="{Binding Reindeer,
Converter={StaticResource EnumToBooleanConverter},
ConverterParameter={x:Static vm:Reindeer.Blitzen}}" />
There you go!
Here's the WPF project: U2UConsult.WPF.DataBindingToEnum.zip (41,39 kb)
Enjoy!