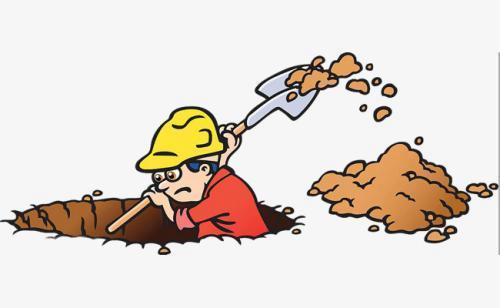
Currently I am doing some consultancy and got asked to port a Web API to .NET 6 which uses JWT tokens for authorization.
In .NET 6 using JWT tokens is quite easy, especially when in combination with Azure AD.
Here is my configuration for dependency injection:
var azureAdSection = builder.Configuration.GetSection("AzureAd");
builder.Services.AddAuthentication(JwtBearerDefaults.AuthenticationScheme)
.AddMicrosoftIdentityWebApi(azureAdSection);
Still every time my access token from the client got rejected. Why?
I started searching the web but could not find anything up to date.
Then my eye saw that in the AddAuthentication
method you can pass a bool
:
var azureAdSection = builder.Configuration.GetSection("AzureAd");
builder.Services.AddAuthentication(JwtBearerDefaults.AuthenticationScheme)
.AddMicrosoftIdentityWebApi(azureAdSection,
subscribeToJwtBearerMiddlewareDiagnosticsEvents: true);
Running this told my my audience was wrong. But what was the expected value?
Seems you can also tell logging to reveal the values of the tokens with:
var azureAdSection = builder.Configuration.GetSection("AzureAd");
builder.Services.AddAuthentication(JwtBearerDefaults.AuthenticationScheme)
.AddMicrosoftIdentityWebApi(azureAdSection,
subscribeToJwtBearerMiddlewareDiagnosticsEvents: true);
IdentityModelEventSource.ShowPII = true;
Running the WebAPI would log everything in the console, even telling me about
the expected value for the audience. Nice!
Hope this helps you with debugging JWT tokens in .NET 6!