Prism 4.0, Managed Extensibility Framework, and Silverlight 4.0 provide an ideal combination to develop robust and flexible enterprise applications. This article explains how you can keep the Prism module catalog in such an application on the server side. In most of the Prism Quickstarts, the module catalog is embedded in the shell:
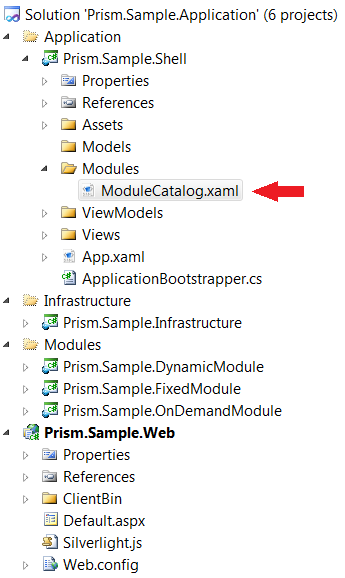
The application class starts the bootstrapper:
private void Application_Startup(
object sender,
StartupEventArgs e)
{
ApplicationBootstrapper bootstrapper = new ApplicationBootstrapper();
bootstrapper.Run();
}
The bootstrapper builds the catalog from the local file:
protected override IModuleCatalog CreateModuleCatalog()
{
ModuleCatalog catalog = Microsoft.Practices.Prism.Modularity.ModuleCatalog.CreateFromXaml(new Uri("Prism.Sample.Shell;component/Modules/ModuleCatalog.xaml", UriKind.Relative));
return catalog;
}
Unfortunately, this setup requires a rebuild and a redeploy of the shell application whenever a change in the catalog is needed (e.g. to add an extra module, or to remove a buggy module). In most -if not all- real-world scenarios it makes more sense to place the catalog in an area that allows easy modification. The following solution has the catalog in a file on the server side, but you can easily go for other storage locations, like a database table, a WCF service or a SharePoint list. Here's where the file is kept:
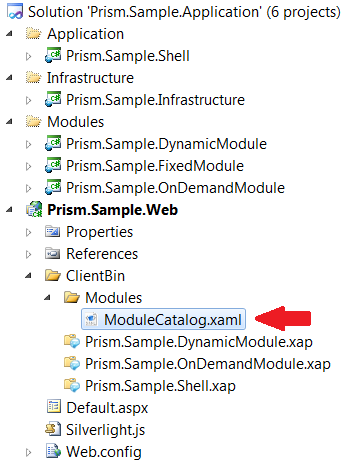
The bootstrapper gets an extra constructor that takes a stream:
private Stream stream;
public ApplicationBootstrapper(Stream stream)
{
this.stream = stream;
}
This stream is used to build the catalog:
protected override IModuleCatalog CreateModuleCatalog()
{
ModuleCatalog catalog = Microsoft.Practices.Prism.Modularity.ModuleCatalog.CreateFromXaml(this.stream);
return catalog;
}
On application startup, the file is read, wrapped in a stream, and handed over to the bootstrapper:
private void Application_Startup(
object sender,
StartupEventArgs e)
{
WebClient client = new WebClient();
client.OpenReadCompleted += this.Client_OpenReadCompleted;
client.OpenReadAsync(new System.Uri(@"Modules\ModuleCatalog.xaml", System.UriKind.Relative));
}
private void Client_OpenReadCompleted(object sender, OpenReadCompletedEventArgs e)
{
ApplicationBootstrapper bootstrapper = new ApplicationBootstrapper(e.Result);
bootstrapper.Run();
}
The following two projects demonstrate both approaches. The application is a small Prism-MEF-Silverlight tutorial application that I wrote a couple of months ago. Here's how it looks like:
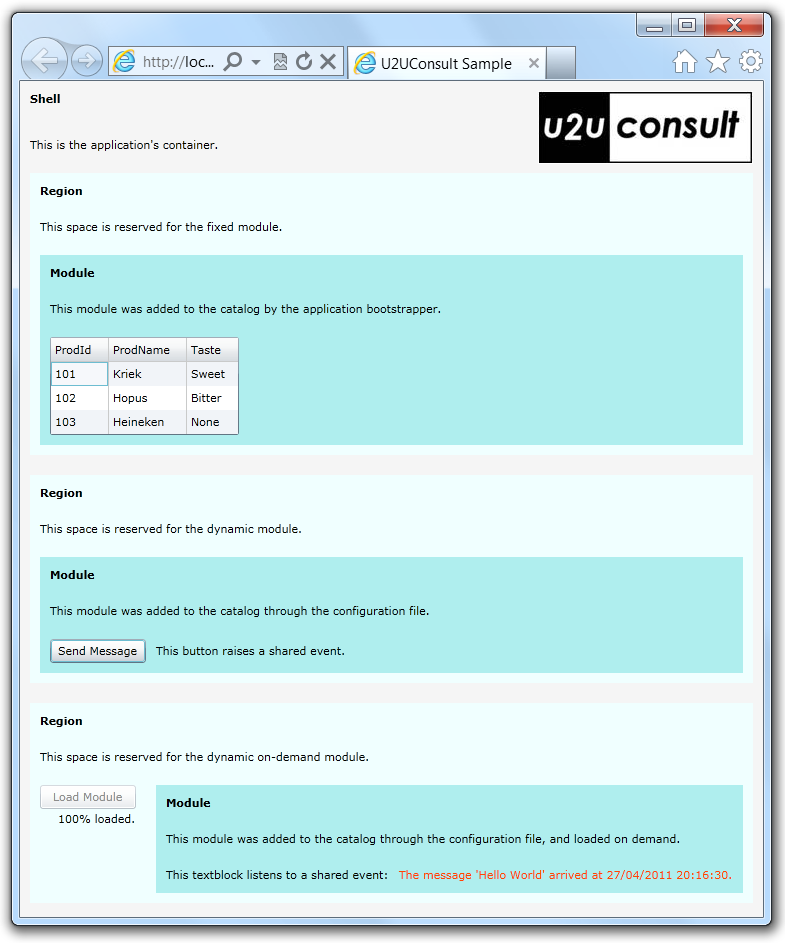
Here's the project before and after the modification (it contains the Prism dll's, so you might need to unblock these):
Enjoy !