The Microsoft WPF Ribbon control is a free control that brings the Office 2007 Ribbon features to your WPF applications. To get your hands on it, just follow the instructions on CodePlex. This article walks through the Ribbon features. I simply built "NotePad with a Ribbon". Here's how it looks like:
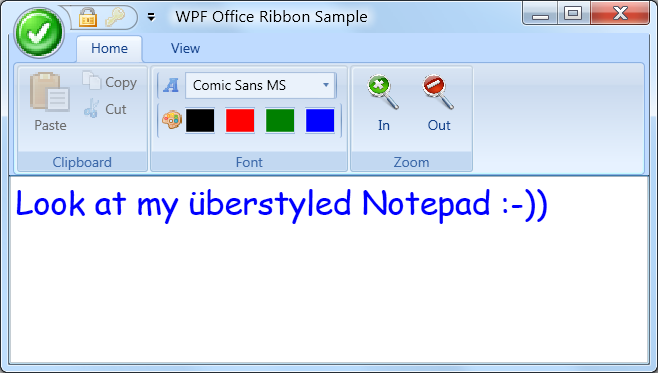
The container is of the type RibbonWindow, a subclass of Window that overrides some of the native GDI-code to let the ApplicationButton and the QuickAccessToolBar appear in the form's title bar. The only controls on it are a Ribbon control and a TextBox (after all, Notepad ìs just a textbox with a menu). I wrapped them in a DockPanel:
<r:RibbonWindow
x:Class="RibbonSample.RibbonSampleWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:r="clr-namespace:Microsoft.Windows.Controls.Ribbon;assembly=RibbonControlsLibrary"
Title="WPF Office Ribbon Sample">
<DockPanel>
<r:Ribbon
DockPanel.Dock="Top"
Title="{Binding RelativeSource={RelativeSource FindAncestor,AncestorType={x:Type Window}},Path=Title}">
</r:Ribbon>
<TextBox
x:Name="NotePadTextBox"
DockPanel.Dock="Top"
AcceptsReturn="True"
AcceptsTab="True"
TextWrapping="Wrap" />
</DockPanel>
</r:RibbonWindow>
The meat of the Ribbon is a series of RibbonTabs with a Label and an array of RibbonGroup elements that contain the RibbonControls (RibbonButton, RibbonComboBox), individually or grouped into a RibbonControlGroup:
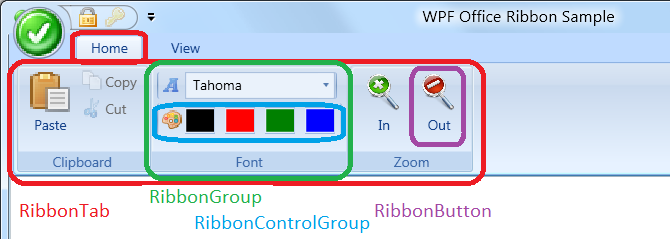
<r:Ribbon
DockPanel.Dock="Top"
Title="{Binding RelativeSource={RelativeSource FindAncestor,AncestorType={x:Type Window}},Path=Title}">
<r:RibbonTab Label="Home">
<r:RibbonTab.Groups>
<!-- Clipboard Commands -->
<r:RibbonGroup>
<r:RibbonGroup.Command>
<r:RibbonCommand LabelTitle="Clipboard" />
</r:RibbonGroup.Command>
<r:RibbonButton Content="Paste" />
<r:RibbonButton Content="Copy" />
<r:RibbonButton Content="Cut" />
</r:RibbonGroup>
<!-- Font Commands -->
<r:RibbonGroup>
<r:RibbonGroup.Command>
<r:RibbonCommand LabelTitle="Font" />
</r:RibbonGroup.Command>
<r:RibbonControlGroup Tag="FontFamily" />
<r:RibbonControlGroup Tag="FontColor" />
</r:RibbonGroup>
<!-- Zoom Commands -->
<r:RibbonGroup>
<r:RibbonGroup.Command>
<r:RibbonCommand LabelTitle="Zoom" />
</r:RibbonGroup.Command>
<r:RibbonButton Content="Zoom In" />
<r:RibbonButton Content="Zoom Out" />
</r:RibbonGroup>
</r:RibbonTab.Groups>
</r:RibbonTab>
<r:RibbonTab Label="View" />
</r:Ribbon>
RibbonGroup has a collection of GroupSizeDefinitions that control layout and resizing. This is the configuration of my Clipboard-group, with one large and two small icons. You can put multiple alternatives here; when you resize the form, the Ribbon will layout properly.
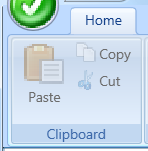
<r:RibbonGroup.GroupSizeDefinitions>
<r:RibbonGroupSizeDefinitionCollection>
<r:RibbonGroupSizeDefinition>
<r:RibbonControlSizeDefinition
ImageSize="Large"
IsLabelVisible="True"/>
<r:RibbonControlSizeDefinition
ImageSize="Small"
IsLabelVisible="True"/>
<r:RibbonControlSizeDefinition
ImageSize="Small"
IsLabelVisible="True"/>
</r:RibbonGroupSizeDefinition>
</r:RibbonGroupSizeDefinitionCollection>
</r:RibbonGroup.GroupSizeDefinitions>
All RibbonControls can have a Command property, and instance of RibbonCommand. This is actually a subclass of RoutedCommand, implementing extra GUI-focused properties: labels, tooltips, and large and small image sources.
<r:RibbonButton Content="Paste">
<r:RibbonButton.Command>
<r:RibbonCommand
CanExecute="PasteCommand_CanExecute"
Executed="PasteCommand_Executed"
LabelTitle="Paste"
ToolTipTitle="Paste"
ToolTipDescription="Paste text element from the clipboard."
SmallImageSource="Assets/Images/Paste.png"
LargeImageSource="Assets/Images/Paste.png" />
</r:RibbonButton.Command>
</r:RibbonButton>
Controls can be (re-)grouped into a RibbonControlGroup e.g. to form a Gallery. I used two such groups for the font commands:
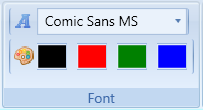
<!-- Font Commands -->
<r:RibbonGroup>
<r:RibbonGroup.Command>
<r:RibbonCommand LabelTitle="Font" />
</r:RibbonGroup.Command>
<r:RibbonControlGroup>
<r:RibbonLabel Tag="Font Image" />
<r:RibbonComboBox>
<r:RibbonComboBoxItem Content="Tahoma"/>
<r:RibbonComboBoxItem Content="Comic Sans MS"/>
<r:RibbonComboBoxItem Content="Wingdings"/>
</r:RibbonComboBox>
</r:RibbonControlGroup>
<r:RibbonControlGroup>
<r:RibbonLabel Tag="Pallette Image" />
<r:RibbonButton CommandParameter="Black" />
<r:RibbonButton CommandParameter="Red" />
<r:RibbonButton CommandParameter="Blue" />
<r:RibbonButton CommandParameter="Green" />
</r:RibbonControlGroup>
</r:RibbonGroup>
Clicking on the Application Button (the large circle in the top left corner) reveals the Ribbon's ApplicationMenu. This can be populated with RibbonApplicationMenuItems, Separators, and RibbonApplicationMenuSplitItems. It also hosts the RecentItemsList. In the ApplicationMenu's footer there's room for extra buttons.
ApplicationMenu |
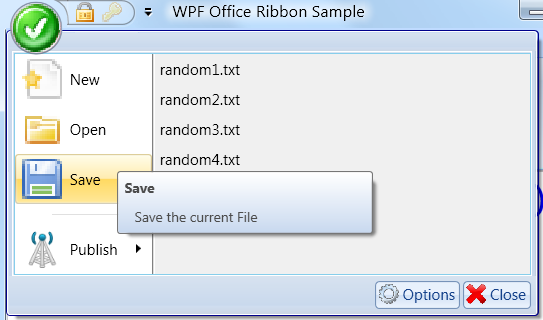 |
RecentItemsList |
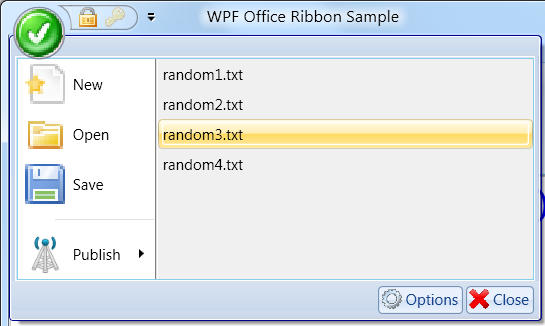 |
ApplicationSplitMenu |
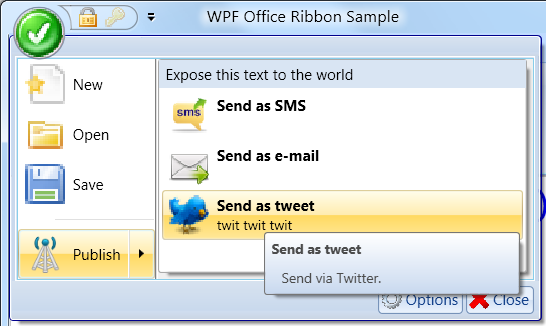 |
<!-- The Application Button -->
<r:Ribbon.ApplicationMenu>
<r:RibbonApplicationMenu>
<r:RibbonApplicationMenu.RecentItemList>
<r:RibbonHighlightingList
MostRecentFileSelected="RibbonHighlightingList_MostRecentFileSelected">
<r:RibbonHighlightingListItem Content="..." />
</r:RibbonHighlightingList>
</r:RibbonApplicationMenu.RecentItemList>
<r:RibbonApplicationMenuItem>
<r:RibbonApplicationMenuItem.Command>
<r:RibbonCommand Executed="FileNew_Executed" />
</r:RibbonApplicationMenuItem.Command>
</r:RibbonApplicationMenuItem>
<Separator />
<r:RibbonApplicationSplitMenuItem>
<r:RibbonApplicationMenuItem>
<r:RibbonApplicationMenuItem.Command>
<r:RibbonCommand Executed="Send_As_SMS" />
</r:RibbonApplicationMenuItem.Command>
</r:RibbonApplicationMenuItem>
<r:RibbonApplicationMenuItem>
<r:RibbonApplicationMenuItem.Command>
<r:RibbonCommand Executed="Send_As_Email" />
</r:RibbonApplicationMenuItem.Command>
</r:RibbonApplicationMenuItem>
</r:RibbonApplicationSplitMenuItem>
<r:RibbonApplicationMenu.Footer>
<DockPanel LastChildFill="False">
<r:RibbonButton DockPanel.Dock="Right" Tag="Close" />
<r:RibbonButton DockPanel.Dock="Right" Tag="Options" />
</DockPanel>
</r:RibbonApplicationMenu.Footer>
</r:RibbonApplicationMenu>
</r:Ribbon.ApplicationMenu>
Yet another host for commands is the QuickAccessToolBar, which can be placed in the form's header next to the ApplicationButton, or under the Ribbon:
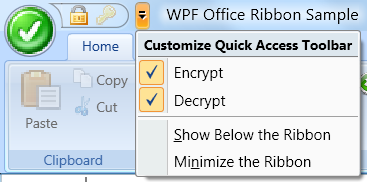
<!-- Quick Access ToolBar -->
<r:Ribbon.QuickAccessToolBar>
<r:RibbonQuickAccessToolBar>
<r:RibbonButton
r:RibbonQuickAccessToolBar.Placement="InCustomizeMenuAndToolBar">
<r:RibbonButton.Command>
<r:RibbonCommand
Executed="EncryptCommand_Executed" />
</r:RibbonButton.Command>
</r:RibbonButton>
<r:RibbonButton
r:RibbonQuickAccessToolBar.Placement="InCustomizeMenuAndToolBar">
<r:RibbonButton.Command>
<r:RibbonCommand
Executed="DecryptCommand_Executed" />
</r:RibbonButton.Command>
</r:RibbonButton>
</r:RibbonQuickAccessToolBar>
</r:Ribbon.QuickAccessToolBar>
If you're going to use Microsoft's WPF Ribbon Control in production, please note that the current release is just a preview, and V1 will have breaking changes in it. On the other hand, a release date hasn't been announced for this V1, all we know is that the control will not be included in .NET 4.0 / Visual Studio.NET 2010.
Here's the full code for the project. Unfortunately I may not publish the full solution, due to licensing constraints on the Ribbon Control. You will need to accept the license, download the ribbon dll yourself, and add a reference.
SOLUTION STRUCTURE
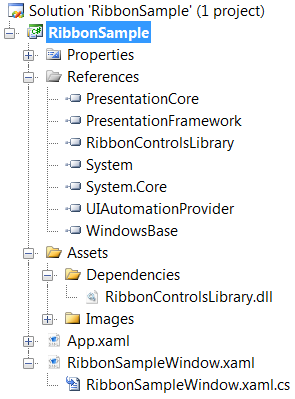
XAML
<r:RibbonWindow x:Class="RibbonSample.RibbonSampleWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:r="clr-namespace:Microsoft.Windows.Controls.Ribbon;assembly=RibbonControlsLibrary"
xmlns:local="clr-namespace:RibbonSample"
Title="WPF Office Ribbon Sample"
Icon="/RibbonSample;component/Assets/Images/dotbay.png">
<Window.Resources>
<ResourceDictionary>
<ResourceDictionary.MergedDictionaries>
<ResourceDictionary Source="/RibbonControlsLibrary;component/Themes/Office2007Blue.xaml" />
</ResourceDictionary.MergedDictionaries>
</ResourceDictionary>
</Window.Resources>
<DockPanel>
<r:Ribbon
DockPanel.Dock="Top"
Title="{Binding RelativeSource={RelativeSource FindAncestor,AncestorType={x:Type Window}},Path=Title}"
>
<!-- The Application Button -->
<r:Ribbon.ApplicationMenu>
<r:RibbonApplicationMenu>
<r:RibbonApplicationMenu.Command>
<r:RibbonCommand
LabelTitle="Application Button"
LabelDescription="Close the application."
ToolTipTitle="WPF Office Ribbon Sample"
ToolTipDescription="Überstyled notepad."
SmallImageSource="/RibbonSample;component/Assets/Images/dotbay.png"
LargeImageSource="/RibbonSample;component/Assets/Images/dotbay.png"/>
</r:RibbonApplicationMenu.Command>
<r:RibbonApplicationMenu.RecentItemList>
<r:RibbonHighlightingList MostRecentFileSelected="RibbonHighlightingList_MostRecentFileSelected">
<r:RibbonHighlightingListItem Content="random1.txt" />
<r:RibbonHighlightingListItem Content="random2.txt" />
<r:RibbonHighlightingListItem Content="random3.txt" />
<r:RibbonHighlightingListItem Content="random4.txt" />
</r:RibbonHighlightingList>
</r:RibbonApplicationMenu.RecentItemList>
<r:RibbonApplicationMenuItem>
<r:RibbonApplicationMenuItem.Command>
<r:RibbonCommand
Executed="FileNew_Executed"
LabelTitle="New"
ToolTipTitle="New"
ToolTipDescription="Create a new File"
LargeImageSource="/RibbonSample;component/Assets/Images/FileNew.png"
SmallImageSource="/RibbonSample;component/Assets/Images/FileNew.png" />
</r:RibbonApplicationMenuItem.Command>
</r:RibbonApplicationMenuItem>
<r:RibbonApplicationMenuItem>
<r:RibbonApplicationMenuItem.Command>
<r:RibbonCommand
Executed="FileOpen_Executed"
LabelTitle="Open"
ToolTipTitle="Open"
ToolTipDescription="Open an existing File"
LargeImageSource="/RibbonSample;component/Assets/Images/FileOpen.png"
SmallImageSource="/RibbonSample;component/Assets/Images/FileOpen.png" />
</r:RibbonApplicationMenuItem.Command>
</r:RibbonApplicationMenuItem>
<r:RibbonApplicationMenuItem>
<r:RibbonApplicationMenuItem.Command>
<r:RibbonCommand
CanExecute="CanAlwaysExecute"
Executed="FileSave_Executed"
LabelTitle="Save"
ToolTipTitle="Save"
ToolTipDescription="Save the current File"
LargeImageSource="/RibbonSample;component/Assets/Images/FileSave.png"
SmallImageSource="/RibbonSample;component/Assets/Images/FileSave.png" />
</r:RibbonApplicationMenuItem.Command>
</r:RibbonApplicationMenuItem>
<Separator />
<r:RibbonApplicationSplitMenuItem>
<r:RibbonApplicationSplitMenuItem.Command>
<r:RibbonCommand
Executed="Not_Yet_Implemented"
LabelTitle="Publish"
LabelDescription="Expose this text to the world"
ToolTipTitle="Publish"
ToolTipDescription="Choose your technology."
SmallImageSource="Assets/Images/publish.png"
LargeImageSource="Assets/Images/publish.png" />
</r:RibbonApplicationSplitMenuItem.Command>
<r:RibbonApplicationMenuItem>
<r:RibbonApplicationMenuItem.Command>
<r:RibbonCommand
Executed="Not_Yet_Implemented"
LabelTitle="Send as SMS"
ToolTipTitle="Send as SMS"
ToolTipDescription="Send as an SMS."
SmallImageSource="Assets/Images/send_sms.png"
LargeImageSource="Assets/Images/send_sms.png" />
</r:RibbonApplicationMenuItem.Command>
</r:RibbonApplicationMenuItem>
<r:RibbonApplicationMenuItem>
<r:RibbonApplicationMenuItem.Command>
<r:RibbonCommand
Executed="Not_Yet_Implemented"
LabelTitle="Send as e-mail"
ToolTipTitle="Send as mail"
ToolTipDescription="Send as an e-mail."
SmallImageSource="Assets/Images/send_mail.png"
LargeImageSource="Assets/Images/send_mail.png" />
</r:RibbonApplicationMenuItem.Command>
</r:RibbonApplicationMenuItem>
<r:RibbonApplicationMenuItem>
<r:RibbonApplicationMenuItem.Command>
<r:RibbonCommand
Executed="Not_Yet_Implemented"
LabelTitle="Send as tweet"
LabelDescription="twit twit twit"
ToolTipTitle="Send as tweet"
ToolTipDescription="Send via Twitter."
SmallImageSource="Assets/Images/send_tweet.png"
LargeImageSource="Assets/Images/send_tweet.png" />
</r:RibbonApplicationMenuItem.Command>
</r:RibbonApplicationMenuItem>
</r:RibbonApplicationSplitMenuItem>
<r:RibbonApplicationMenu.Footer>
<DockPanel LastChildFill="False">
<r:RibbonButton
Margin="2"
DockPanel.Dock="Right"
>
<r:RibbonButton.Command>
<r:RibbonCommand
Executed="Not_Yet_Implemented"
LabelTitle="Close"
ToolTipTitle="Close"
ToolTipDescription="Close the Application Menu."
SmallImageSource="Assets/Images/Exit.png"
LargeImageSource="Assets/Images/Exit.png" />
</r:RibbonButton.Command>
</r:RibbonButton>
<r:RibbonButton
DockPanel.Dock="Right"
>
<r:RibbonButton.Command>
<r:RibbonCommand
Executed="Not_Yet_Implemented"
LabelTitle="Options"
ToolTipTitle="Options"
ToolTipDescription="Extra options."
SmallImageSource="Assets/Images/Configuration.png"
LargeImageSource="Assets/Images/Configuration.png" />
</r:RibbonButton.Command>
</r:RibbonButton>
</DockPanel>
</r:RibbonApplicationMenu.Footer>
</r:RibbonApplicationMenu>
</r:Ribbon.ApplicationMenu>
<!-- Quick Access ToolBar -->
<r:Ribbon.QuickAccessToolBar>
<r:RibbonQuickAccessToolBar>
<r:RibbonButton
r:RibbonQuickAccessToolBar.Placement="InCustomizeMenuAndToolBar">
<r:RibbonButton.Command>
<r:RibbonCommand
CanExecute="EncryptCommand_CanExecute"
Executed="EncryptCommand_Executed"
LabelTitle="Encrypt"
ToolTipTitle="Encrypt"
ToolTipDescription="Clear all text."
SmallImageSource="Assets/Images/Encrypt.png"
LargeImageSource="Assets/Images/Encrypt.png" />
</r:RibbonButton.Command>
</r:RibbonButton>
<r:RibbonButton
r:RibbonQuickAccessToolBar.Placement="InCustomizeMenuAndToolBar">
<r:RibbonButton.Command>
<r:RibbonCommand
CanExecute="DecryptCommand_CanExecute"
Executed="DecryptCommand_Executed"
LabelTitle="Decrypt"
ToolTipTitle="Decrypt"
ToolTipDescription="Uncipher the text."
SmallImageSource="Assets/Images/Decrypt.png"
LargeImageSource="Assets/Images/Decrypt.png" />
</r:RibbonButton.Command>
</r:RibbonButton>
</r:RibbonQuickAccessToolBar>
</r:Ribbon.QuickAccessToolBar>
<r:RibbonTab Label="Home">
<r:RibbonTab.Groups>
<!-- Clipboard Commands -->
<r:RibbonGroup>
<r:RibbonGroup.GroupSizeDefinitions>
<r:RibbonGroupSizeDefinitionCollection>
<r:RibbonGroupSizeDefinition>
<r:RibbonControlSizeDefinition
ImageSize="Large"
IsLabelVisible="True"/>
<r:RibbonControlSizeDefinition
ImageSize="Small"
IsLabelVisible="True"/>
<r:RibbonControlSizeDefinition
ImageSize="Small"
IsLabelVisible="True"/>
</r:RibbonGroupSizeDefinition>
</r:RibbonGroupSizeDefinitionCollection>
</r:RibbonGroup.GroupSizeDefinitions>
<r:RibbonGroup.Command>
<r:RibbonCommand LabelTitle="Clipboard" />
</r:RibbonGroup.Command>
<r:RibbonButton Content="Paste">
<r:RibbonButton.Command>
<r:RibbonCommand
CanExecute="PasteCommand_CanExecute"
Executed="PasteCommand_Executed"
LabelTitle="Paste"
ToolTipTitle="Paste"
ToolTipDescription="Paste text element from the clipboard."
SmallImageSource="Assets/Images/Paste.png"
LargeImageSource="Assets/Images/Paste.png" />
</r:RibbonButton.Command>
</r:RibbonButton>
<r:RibbonButton Content="Copy">
<r:RibbonButton.Command>
<r:RibbonCommand
CanExecute="CopyCommand_CanExecute"
Executed="CopyCommand_Executed"
LabelTitle="Copy"
ToolTipTitle="Copy"
ToolTipDescription="Copy text element to the clipboard."
SmallImageSource="Assets/Images/Copy.png"
LargeImageSource="Assets/Images/Copy.png" />
</r:RibbonButton.Command>
</r:RibbonButton>
<r:RibbonButton Content="Cut">
<r:RibbonButton.Command>
<r:RibbonCommand
CanExecute="CutCommand_CanExecute"
Executed="CutCommand_Executed"
LabelTitle="Cut"
ToolTipTitle="Cut"
ToolTipDescription="Cut text element to the clipboard."
SmallImageSource="Assets/Images/Cut.png"
LargeImageSource="Assets/Images/Cut.png" />
</r:RibbonButton.Command>
</r:RibbonButton>
</r:RibbonGroup>
<!-- Font Commands -->
<r:RibbonGroup>
<r:RibbonGroup.GroupSizeDefinitions>
<r:RibbonGroupSizeDefinitionCollection>
<r:RibbonGroupSizeDefinition>
<r:RibbonControlSizeDefinition
ImageSize="Small"
IsLabelVisible="True"/>
<r:RibbonControlSizeDefinition
ImageSize="Small"
IsLabelVisible="True"/>
</r:RibbonGroupSizeDefinition>
</r:RibbonGroupSizeDefinitionCollection>
</r:RibbonGroup.GroupSizeDefinitions>
<r:RibbonGroup.Command>
<r:RibbonCommand LabelTitle="Font" />
</r:RibbonGroup.Command>
<r:RibbonControlGroup Margin=" 0 3 0 3">
<r:RibbonLabel
Padding="0 3" Margin="3 0"
VerticalAlignment="Bottom">
<r:RibbonLabel.Content>
<Image
Margin="0" Height="16"
Source="/RibbonSample;component/Assets/Images/Font.png" />
</r:RibbonLabel.Content>
</r:RibbonLabel>
<r:RibbonComboBox
x:Name="FontComboBox"
SelectionChanged="FontComboBox_SelectionChanged"
SelectedIndex="0"
MinWidth="120"
IsReadOnly="True"
IsEditable="True">
<r:RibbonComboBoxItem Content="Tahoma"/>
<r:RibbonComboBoxItem Content="Comic Sans MS"/>
<r:RibbonComboBoxItem Content="Wingdings"/>
</r:RibbonComboBox>
</r:RibbonControlGroup>
<r:RibbonControlGroup Height="28">
<r:RibbonLabel
Padding="0 6" Margin="3 0" VerticalAlignment="Bottom">
<r:RibbonLabel.Content>
<Image
Margin="0" Height="16"
Source="/RibbonSample;component/Assets/Images/Palette.png" />
</r:RibbonLabel.Content>
</r:RibbonLabel>
<r:RibbonButton
CommandParameter="Black"
ToolTip="Black"
Background="Black"
Margin="0 3 4 3">
<r:RibbonButton.Command>
<r:RibbonCommand
Executed="FontColorCommand_Executed"/>
</r:RibbonButton.Command>
</r:RibbonButton>
<r:RibbonButton
CommandParameter="Red"
ToolTip="Red"
Background="Red"
Margin="4 3">
<r:RibbonButton.Command>
<r:RibbonCommand
Executed="FontColorCommand_Executed"/>
</r:RibbonButton.Command>
</r:RibbonButton>
<r:RibbonButton
CommandParameter="Green"
ToolTip="Green"
Background="Green"
Margin="4 3">
<r:RibbonButton.Command>
<r:RibbonCommand
Executed="FontColorCommand_Executed"/>
</r:RibbonButton.Command>
</r:RibbonButton>
<r:RibbonButton
CommandParameter="Blue"
ToolTip="Blue"
Background="Blue"
Margin="4 3">
<r:RibbonButton.Command>
<r:RibbonCommand
Executed="FontColorCommand_Executed"/>
</r:RibbonButton.Command>
</r:RibbonButton>
</r:RibbonControlGroup>
</r:RibbonGroup>
<!-- Zoom Commands -->
<r:RibbonGroup>
<r:RibbonGroup.GroupSizeDefinitions>
<r:RibbonGroupSizeDefinitionCollection>
<r:RibbonGroupSizeDefinition>
<r:RibbonControlSizeDefinition
ImageSize="Large"
IsLabelVisible="True"/>
<r:RibbonControlSizeDefinition
ImageSize="Large"
IsLabelVisible="True"/>
</r:RibbonGroupSizeDefinition>
</r:RibbonGroupSizeDefinitionCollection>
</r:RibbonGroup.GroupSizeDefinitions>
<r:RibbonGroup.Command>
<r:RibbonCommand LabelTitle="Zoom" />
</r:RibbonGroup.Command>
<r:RibbonButton Content="Zoom In">
<r:RibbonButton.Command>
<r:RibbonCommand
Executed="ZoomInCommand_Executed"
LabelTitle="In"
ToolTipTitle="Zoom In"
ToolTipDescription="Zoom In."
SmallImageSource="Assets/Images/Zoom-in.png"
LargeImageSource="Assets/Images/Zoom-in.png" />
</r:RibbonButton.Command>
</r:RibbonButton>
<r:RibbonButton Content="Zoom Out">
<r:RibbonButton.Command>
<r:RibbonCommand
CanExecute="ZoomOutCommand_CanExecute"
Executed="ZoomOutCommand_Executed"
LabelTitle="Out"
ToolTipTitle="Zoom Out"
ToolTipDescription="Zoom Out."
SmallImageSource="Assets/Images/Zoom-out.png"
LargeImageSource="Assets/Images/Zoom-out.png" />
</r:RibbonButton.Command>
</r:RibbonButton>
</r:RibbonGroup>
</r:RibbonTab.Groups>
</r:RibbonTab>
<r:RibbonTab Label="View">
</r:RibbonTab>
</r:Ribbon>
<TextBox
x:Name="NotePadTextBox"
DockPanel.Dock="Top"
AcceptsReturn="True"
AcceptsTab="True"
TextWrapping="Wrap"
/>
</DockPanel>
</r:RibbonWindow>
C#
namespace RibbonSample
{
using System;
using System.IO;
using System.Text;
using System.Windows.Input;
using System.Windows.Media;
using Microsoft.Windows.Controls.Ribbon;
using Microsoft.Win32;
public partial class RibbonSampleWindow : RibbonWindow
{
public RibbonSampleWindow()
{
InitializeComponent();
this.IsEncrypted = false;
// Programmatically setting the skin:
// this.Resources.MergedDictionaries.Add(PopularApplicationSkins.Office2007Blue);
this.NotePadTextBox.Focus();
}
public bool IsEncrypted { get; set; }
public string FileName { get; set; }
#region Quick Access Toolbar Commands
private void EncryptCommand_CanExecute(object sender, CanExecuteRoutedEventArgs e)
{
e.CanExecute = !this.IsEncrypted;
}
private void EncryptCommand_Executed(object sender, ExecutedRoutedEventArgs e)
{
// No real Rijndael, just obfuscation !!!
this.NotePadTextBox.Text =
Convert.ToBase64String(ASCIIEncoding.ASCII.GetBytes(this.NotePadTextBox.Text));
this.NotePadTextBox.IsEnabled = false;
this.IsEncrypted = true;
}
private void DecryptCommand_CanExecute(object sender, CanExecuteRoutedEventArgs e)
{
e.CanExecute = this.IsEncrypted;
}
private void DecryptCommand_Executed(object sender, ExecutedRoutedEventArgs e)
{
this.NotePadTextBox.Text =
ASCIIEncoding.ASCII.GetString(Convert.FromBase64String(this.NotePadTextBox.Text));
this.NotePadTextBox.IsEnabled = true;
this.IsEncrypted = false;
}
#endregion
#region Application Button Commands
private void RibbonHighlightingList_MostRecentFileSelected(object sender, MostRecentFileSelectedEventArgs e)
{
this.FileName = (string)e.SelectedItem;
this.Title = this.FileName;
}
private void FileNew_Executed(object sender, ExecutedRoutedEventArgs e)
{
this.NotePadTextBox.Text = string.Empty;
this.FileName = "New Document.txt";
this.Title = this.FileName;
}
private void FileOpen_Executed(object sender, ExecutedRoutedEventArgs e)
{
OpenFileDialog dlg = new OpenFileDialog();
dlg.FileName = "Document";
dlg.DefaultExt = ".txt";
dlg.Filter = "Text documents (.txt)|*.txt";
if (dlg.ShowDialog() == true)
{
this.FileName = dlg.FileName;
this.Title = FileName;
this.NotePadTextBox.Text = File.ReadAllText(this.FileName);
}
}
private void FileSave_Executed(object sender, ExecutedRoutedEventArgs e)
{
SaveFileDialog dlg = new SaveFileDialog();
if (!string.IsNullOrEmpty(this.FileName))
{
dlg.FileName = this.FileName;
}
else
{
dlg.FileName = "Document";
}
dlg.DefaultExt = ".text";
dlg.Filter = "Text documents (.txt)|*.txt";
if (dlg.ShowDialog() == true)
{
FileName = dlg.FileName;
this.Title = FileName;
File.WriteAllText(FileName, this.NotePadTextBox.Text);
}
}
#endregion
#region ClipBoard Commands
private void CopyCommand_CanExecute(object sender, CanExecuteRoutedEventArgs e)
{
e.CanExecute = ApplicationCommands.Copy.CanExecute(null, null);
}
private void PasteCommand_CanExecute(object sender, CanExecuteRoutedEventArgs e)
{
e.CanExecute = ApplicationCommands.Paste.CanExecute(null, null);
}
private void CutCommand_CanExecute(object sender, CanExecuteRoutedEventArgs e)
{
e.CanExecute = ApplicationCommands.Cut.CanExecute(null, null);
}
private void PasteCommand_Executed(object sender, ExecutedRoutedEventArgs e)
{
ApplicationCommands.Paste.Execute(null, null);
}
private void CopyCommand_Executed(object sender, ExecutedRoutedEventArgs e)
{
ApplicationCommands.Copy.Execute(null, null);
}
private void CutCommand_Executed(object sender, ExecutedRoutedEventArgs e)
{
ApplicationCommands.Cut.Execute(null, null);
}
#endregion
#region Font Commands
private void FontComboBox_SelectionChanged(object sender, System.Windows.Controls.SelectionChangedEventArgs e)
{
if (this.NotePadTextBox != null)
{
switch (this.FontComboBox.SelectedIndex)
{
case 0:
this.NotePadTextBox.FontFamily = new FontFamily("Tahoma");
return;
case 1:
this.NotePadTextBox.FontFamily = new FontFamily("Comic Sans MS");
return;
case 2:
this.NotePadTextBox.FontFamily = new FontFamily("Wingdings");
return;
default:
return;
}
}
}
private void FontColorCommand_Executed(object sender, ExecutedRoutedEventArgs e)
{
this.NotePadTextBox.Foreground =
new SolidColorBrush(
(Color)ColorConverter.ConvertFromString(e.Parameter as string));
}
#endregion
#region Zoom Commands
private void ZoomInCommand_Executed(object sender, ExecutedRoutedEventArgs e)
{
this.NotePadTextBox.FontSize++;
}
private void ZoomOutCommand_CanExecute(object sender, CanExecuteRoutedEventArgs e)
{
e.CanExecute = (this.NotePadTextBox.FontSize > 1);
}
private void ZoomOutCommand_Executed(object sender, ExecutedRoutedEventArgs e)
{
this.NotePadTextBox.FontSize--;
}
#endregion
#region Helper Commands
private void CanAlwaysExecute(object sender, CanExecuteRoutedEventArgs e)
{
e.CanExecute = true;
}
private void Not_Yet_Implemented(object sender, ExecutedRoutedEventArgs e)
{
// Not yet implemented ...
}
#endregion
}
}