These days, you don't need a Program
class with a static void Main()
anymore. You can just write code directly in Program.cs
.
This is called using top-level statements. However, you might run into a couple of surprises when writing code here.
For example, try writing the following functions in Program.cs
:
Program.cs
void Print(int value) => Console.WriteLine(value);
void Print(string value) => Console.WriteLine(value);
This will give you the following exception:
A local variable named 'Print' is already defined in this scope
It seems that overloading is not allowed wile using top-level statements. But why is that?
Investigation
What you need to realize, is that top-level statements are just syntactic sugar. There is still a Program
class with a Main()
method.
You can see this by inspecting your compiled code with something like ildasm
.
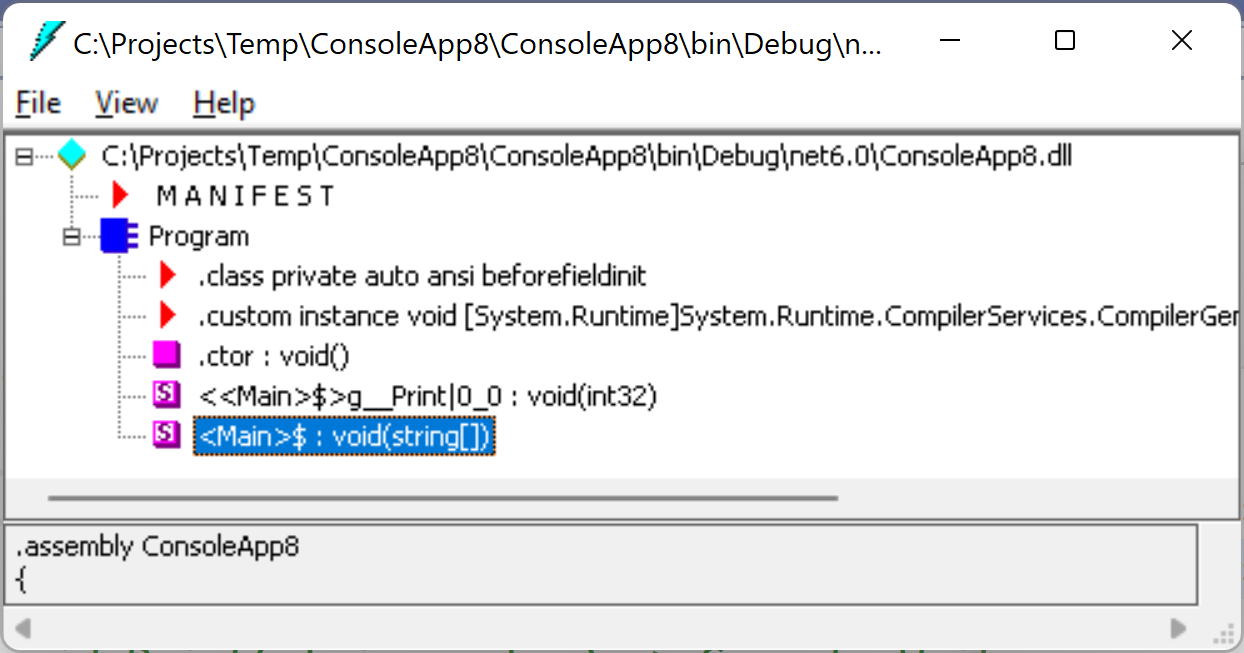
What you are writing in Program.cs
is in fact the content of the Main()
method.
That means the Print functions are local functions. They are defined within the declaration space of a function, not of a type (class, struct, ...).
Currently overloading is only allowed within the declaration space of a type.
It is possible that one day overloading with local functions will be allowed, but not today.
The (Somewhat of a) Solution
If you really want to define multiple local functions with the same name, you can just use a local scope. This isn't very practical though because the call to the function needs to be in the same scope.
Program.cs
{
Print(7);
void Print(int value) => Console.WriteLine(value);
}
{
Print("Seven");
void Print(string value) => Console.WriteLine(value);
}
Conclusion
Today it's not possible to use overloading in Program.cs
. This might change in the future. However, does it really matter?
You are effectively working with local functions; you can't access them from outside Program.cs
. And if this is just a local effect, how bad is it to just name your functions PrintInt
and PrintString
?