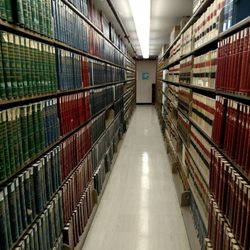
Domain Driven Design uses a series of patterns for building applications that are more maintainable,
and allow a complex application to stand the test of time. One of these patterns is Repository,
and a lot of people spend time building their own repositories.
[More]
Summary: This post is about how to enable migrations for library projects, with user-secrets.
[More]