In this blog post I will illustrate how you can build an SPFx solution that will open up a panel at the side when clicking a button in the commandbar in SharePoint Online. I'll illustrate this using React components, but it will be similar using other frameworks.
With the introduction of CommandSet in SPFx, it is possible to add your custom buttons to the command bar in SharePoint Online. If you already played with the sample generated by Yeoman or had a look at the samples on the internet, you will observe that most samples stick to using the Dialog. Now, a lot of existing actions in a modern SharePoint Online site pop up that pane at the right. What if you want this as well?
Use yeoman to generate a default commandset SPFx project. I'll use the Panel from Office UI Fabric and create my own React component around it, not that it is mandatory, but it will give me more control later on ...
The code for my component:
import * as React from 'react';
import { Panel, PanelType } from 'office-ui-fabric-react/lib/Panel';
export default class CustomPanel extends React.Component<{}, {}> {
public render(): React.ReactElement<{}> {
return (
<div>
<Panel
isOpen={true}
type={ PanelType.smallFixedFar }
headerText='U2U Panel'
closeButtonAriaLabel='Close'
>
<p>Hello there!</p>
</Panel>
</div>
);
}
}
Now the trick is to make sure that when a button is clicked, that you create a div element inside your page in which you can then render your panel. You can do this inside the onExecute of the default generated code for your CommandSet. The code that does the trick looks as follows:
public onExecute(event: IListViewCommandSetExecuteEventParameters): void {
switch (event.itemId) {
...
case 'COMMAND_2':
const div = document.createElement('div');
const element: React.ReactElement<{} > = React.createElement(
CustomPanel,
{
}
);
ReactDom.render(element, div);
break;
default:
throw new Error('Unknown command');
}
}
The result will look as follows:
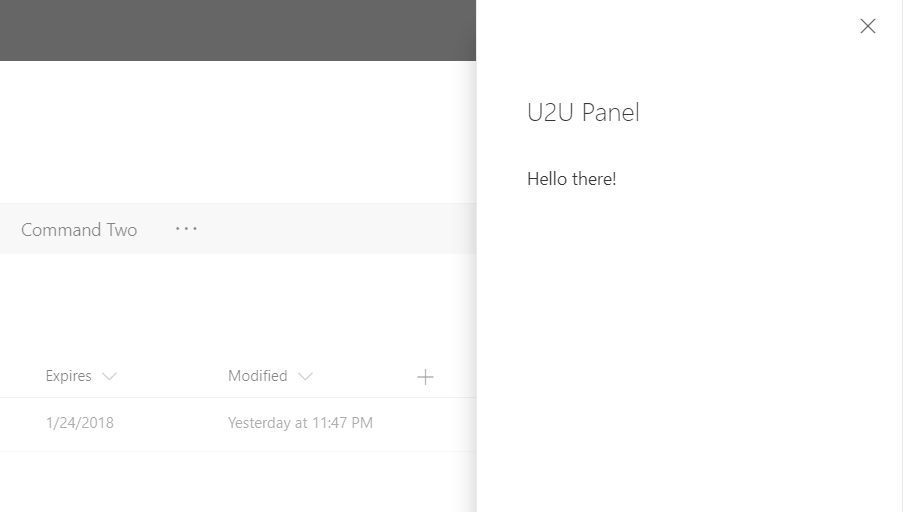